Check if a String Contains a Substring in JavaScript
There's two common ways to check whether a string contains a substring in JavaScript. The more modern way is the String#includes()
function.
const str = 'Arya Stark';
str.includes('Stark'); // true
str.includes('Snow'); // false
You can use String#includes()
in all modern browsers except Internet Explorer. You can also use String#includes()
in Node.js >= 4.0.0
.
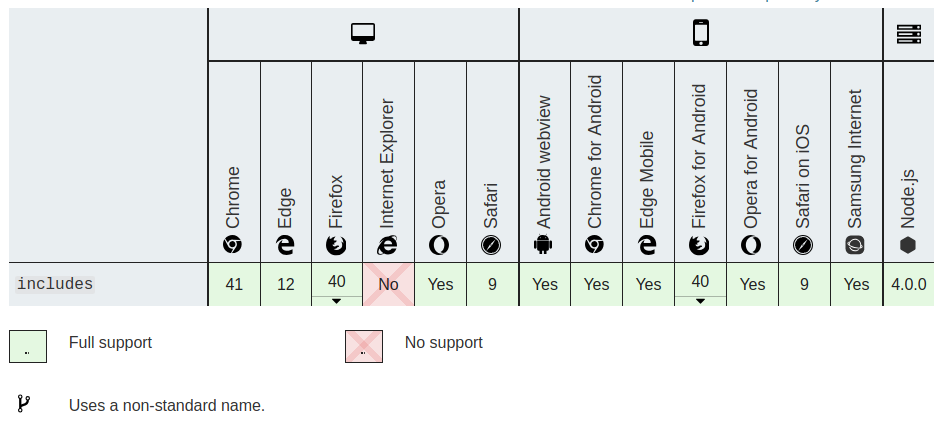
Compatibility table from Mozilla Developer Network
If you need to support Internet Explorer, you should instead use the String#indexOf()
method, which has been a part of JavaScript since ES1 in 1997.
const str = 'Arya Stark';
str.indexOf('Stark') !== -1; // true
str.indexOf('Snow') !== -1; // false
In general, if you have any doubt about whether code will run in an environment that supports includes()
, you should use indexOf()
. The includes()
function's syntax is only marginally more concise than indexOf()
.
Case Insensitive Search
Both String#includes()
and String#indexOf()
are case sensitive. Neither function supports regular expressions. To do case insensitive search, you can use regular expressions and the String#match()
function, or you can convert both the string and substring to lower case using the String#toLowerCase()
function.
const str = 'arya stark';
// The most concise way to check substrings ignoring case is using
// `String#match()` and a case-insensitive regular expression (the 'i')
str.match(/Stark/i); // true
str.match(/Snow/i); // false
// You can also convert both the string and the search string to lower case.
str.toLowerCase().includes('Stark'.toLowerCase()); // true
str.toLowerCase().indexOf('Stark'.toLowerCase()) !== -1; // true
str.toLowerCase().includes('Snow'.toLowerCase()); // false
str.toLowerCase().indexOf('Snow'.toLowerCase()) !== -1; // false