The Difference Between in and hasOwnProperty in JavaScript
Given a general JavaScript object, there are two common ways to check whether an object contains a key: the in
operator and the hasOwnProperty()
function. With a simple POJO and no special keys, these two are equivalent:
const obj = { answer: 42 };
'answer' in obj; // true
obj.hasOwnProperty('answer'); // true
'does not exist' in obj; // false
obj.hasOwnProperty('does not exist'); // false
Both also support ES6 symbols.
const symbol = Symbol('answer');
const obj = { [symbol]: 42 };
symbol in obj; // true
obj.hasOwnProperty(symbol); // true
So what's the difference between the two? The key difference is that in
will return true
for inherited properties, whereas hasOwnProperty()
will return false
for inherited properties.
For example, the Object
base class in JavaScript has a __proto__
property, a constructor
property, and a hasOwnProperty
function. The in
operator will return true
for these properties, but hasOwnProperty()
will return false
.
'constructor' in obj; // true
'__proto__' in obj; // true
'hasOwnProperty' in obj; // true
obj.hasOwnProperty('constructor'); // false
obj.hasOwnProperty('__proto__'); // false
obj.hasOwnProperty('hasOwnProperty'); // false
Because hasOwnProperty()
ignores inherited properties, it is the better choice for plain old JavaScript objects (POJOs). However, hasOwnProperty()
will return false
for ES6 class getters and methods, like ES6 getters.
class BaseClass {
get baseProp() {
return 42;
}
}
class ChildClass extends BaseClass {
get childProp() {
return 42;
}
}
const base = new BaseClass();
const child = new ChildClass();
'baseProp' in base; // true
'childProp' in child; // true
'baseProp' in child; // true
base.hasOwnProperty('baseProp'); // false
child.hasOwnProperty('childProp'); // false
child.hasOwnProperty('baseProp'); // false
Below is a summary of the tradeoffs between in
and hasOwnProperty()
.
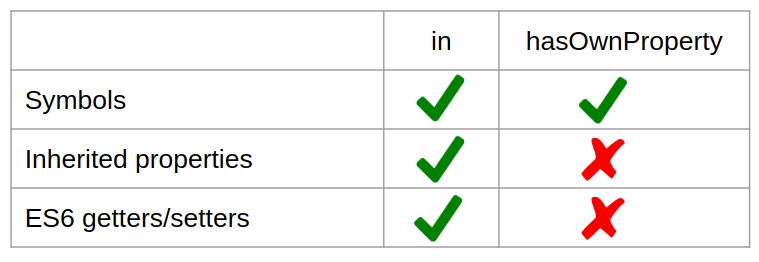
In general, hasOwnProperty()
is the right choice most of the time, because you avoid issues with special keys, like constructor
. A good rule of thumb is that if you're looking to see whether an object has a property, you should use hasOwnProperty()
. If you're looking to see if an object has a function that you intend to call, like checking if an object has toString()
, you should use in
.