How to Use JavaScript's `Promise.allSettled()` Function
Oct 19, 2021
Promise.allSettled()
is similar to Promise.all()
with two key differences:
allSettled()
will resolve regardless of whether one of the promises was rejectedallSettled()
will return an array of objects, as opposed to an array, that contain the{status, value, reason}
that describes whether each promise was fulfilled or rejected.
Recall that a promise is a state machine with 3 states:
- Pending The operation is in progress.
- Fulfilled The operation completed successfully.
- Rejected The operation experienced an error.
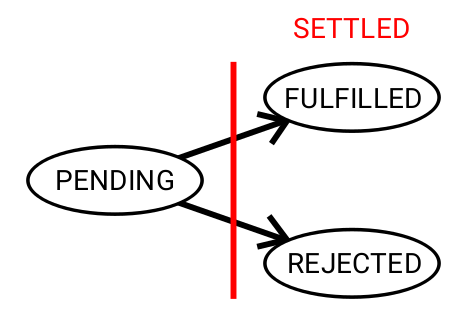
"Settled" means the promise is either fulfilled or rejected, so you can think of allSettled()
as waiting for all the promises in the array to become settled.
Return Value
allSettled()
will contain an array of objects that contain either {status: 'fulfilled', value}
if the promise was fulfilled or {status: 'rejected', reason}
if the promise was rejected.
// [{ status: "fulfilled", value: "Hello World" }, { status: "rejected", reason: "fail" }]
const res = await Promise.allSettled([Promise.resolve('Hello World'), Promise.reject('fail')]);
To check if any promise was rejected, you can use the Array#find()
function:
res.find(({ status }) => status === 'rejected');
Browser Support
allSettled()
is not supported in Internet Explorer and Node.js versions below 12.9.
However, you can use Promise.all()
for environments that do not support allSettled()
as shown below:
function allSettled(promises) {
const _promises = promises.map(p => {
return p.
then(value => ({ status: 'fulfilled', value })).
catch(reason => ({ status: 'rejected', reason });
});
return Promise.all(_promises);
}
Did you find this tutorial useful? Say thanks by starring our repo on GitHub!