How to Locally Scope the CSS in Your Vue Files
Apr 9, 2021
Vue 3 has a handy way to locally scope the CSS in your components.
Using <style scoped>
, you don't need to have a single large CSS
file or multiple CSS files to make your site look pretty. By simply
putting the CSS in the <style scoped>
tag, the CSS will apply to
that component.
App.vue
<template>
<img alt="Vue logo" src="./assets/logo.png" />
<HelloWorld msg="Welcome to Your Vue.js App" />
</template>
<script>
import HelloWorld from "./components/HelloWorld.vue";
export default {
name: "App",
components: {
HelloWorld,
},
};
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</style>
HelloWorld.vue
<template>
<div class="hello">
<h1>{{ msg }}</h1>
<p class="text">This text is in a component with a {{ html }}</p>
</div>
</template>
<script>
export default {
name: "HelloWorld",
data() {
return {
html: `<style scoped>`,
};
},
props: {
msg: String,
},
};
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
h3 {
margin: 40px 0 0;
}
ul {
list-style-type: none;
padding: 0;
}
li {
display: inline-block;
margin: 0 10px;
}
a {
color: #42b983;
}
.text {
color: pink;
}
</style>
Result
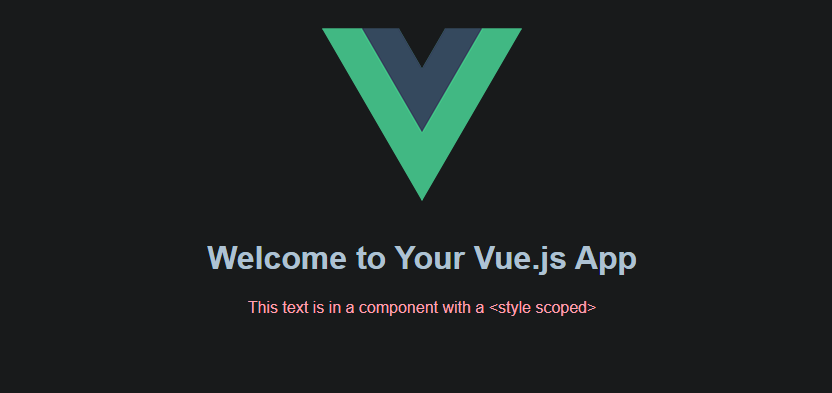
Vue School has some of our favorite Vue
video courses. Their Vue.js Master Class walks you through building a real
world application, and does a great job of teaching you how to integrate Vue
with Firebase.
Check it out!
Did you find this tutorial useful? Say thanks by starring our repo on GitHub!