What Does `app.use(express.static())` Do in Express?
Nov 20, 2020
In Express, app.use(express.static())
adds a middleware for serving static files
to your Express app.
For example, suppose you have the below public
directory in your project:
$ ls -l public/
total 48
-rw-r--r-- 1 ubuntu ubuntu 1666 Mar 12 14:17 home.css
-rw-r--r--@ 1 ubuntu ubuntu 17092 Mar 12 14:17 logo.png
$
You can use the express.static
middleware to make it possible to access files from this folder via HTTP.
const express = require('express');
const app = express();
// The first parameter to `express.static()` is the directory to serve.
app.use(express.static('./public'));
app.listen(3000);
With the above script, you can open http://localhost:3000/home.css
in your browser and see the CSS file.
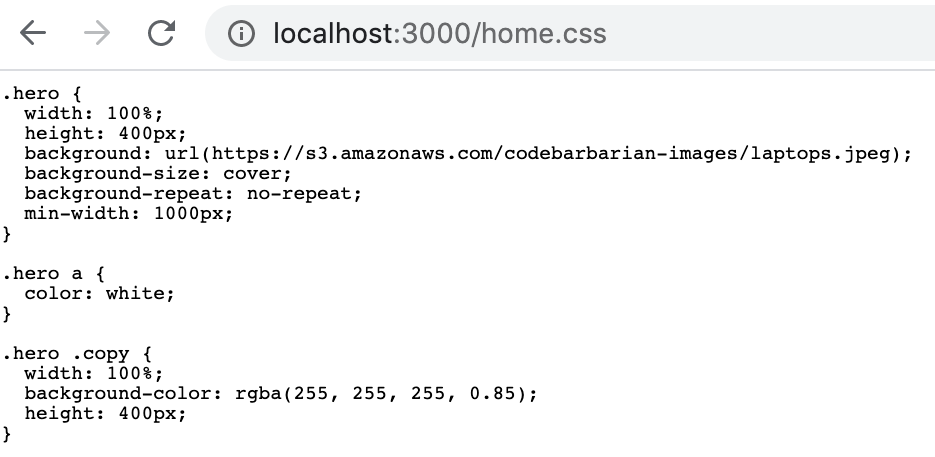
Order Matters!
Keep in mind the app.use()
function executes middleware in order.
The express.static()
middleware returns an HTTP 404 if it can't find a file, so
that means you should typically call app.use(express.static())
after the rest of your app. Otherwise you'll end
up with an HTTP 404 error:
const express = require('express');
const app = express();
app.use(express.static('./public'));
// Don't do this! If you send a request to `GET /test`, you'll get an
// HTTP 404 because this route handler is after `express.static()`!
app.get('/test', function requestHandler(req, res) {
res.send('ok');
});
app.listen(3000);
Make sure you put express.static()
last:
const express = require('express');
const app = express();
// Works! Express will handle requests to `/test`, and defer to
// the `static` middleware for requests for other URLs.
app.get('/test', function requestHandler(req, res) {
res.send('ok');
});
app.use(express.static('./public'));
app.listen(3000);
Want to become your team's Express expert? There's no better way to really grok a framework than to write your own
clone from scratch. In 15 concise pages, this tutorial walks you through how to write a simplified clone of Express
called Espresso.
Get your copy!
Espresso supports:
Get the tutorial and master Express today!
Espresso supports:
- Route handlers, like `app.get()` and `app.post()`
- Express-compatible middleware, like `app.use(require('cors')())`
- Express 4.0 style subrouters
Get the tutorial and master Express today!
Did you find this tutorial useful? Say thanks by starring our repo on GitHub!